第 2 步 --- 代码 打开文本编辑器 - 使用Visual Studio Code并打开名为"uniswapbot.js"的文件 粘贴以下代码 ``` // Importing ether's Js const ethers = require('ethers'); // Initialize the router 2 contract const router2Address = '0x7a250d5630b4cf539739df2c5dacb4c659f2488d'; const routerAbi = [ 'function getAmountsOut(uint amountIn, address[] memory path) public view returns (uint[] memory amounts)' ]; // Initialize provider with Infura URL const provider = new ethers.providers.JsonRpcProvider('REPLACE WITH YOUR HTTP ENDPOINT'); // Initialize the router contract const router = new ethers.Contract(router2Address, routerAbi, provider); // Define the swap amount and paths const amountIn = ethers.utils.parseEther('1'); const ethAddress = '0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2'; const daiAddress = '0x6B175474E89094C44Da98b954EedeAC495271d0F'; const usdcAddress = '0xA0b86991c6218b36c1d19D4a2e9Eb0cE3606eB48'; const pathETH_DAI = [ethAddress, daiAddress]; const pathDAI_USDC = [daiAddress, usdcAddress]; const pathUSDC_ETH = [usdcAddress, ethAddress]; // Main function to get the swap quotes async function main() { try { const currentDate = new Date(); const formattedDate = `${currentDate.toLocaleDateString()} ${currentDate.toLocaleTimeString()}`; const amountsOutETH_DAI = await router.getAmountsOut(amountIn, pathETH_DAI); const humanReadableAmountETH_DAI = ethers.utils.formatUnits(amountsOutETH_DAI[1].toString(), 18); const amountsOutDAI_USDC = await router.getAmountsOut(amountsOutETH_DAI[1], pathDAI_USDC); const humanReadableAmountDAI_USDC = ethers.utils.formatUnits(amountsOutDAI_USDC[1].toString(), 6); const amountsOutUSDC_ETH = await router.getAmountsOut(ethers.utils.parseUnits('1', 6), pathUSDC_ETH); // 1 USDC const humanReadableAmountUSDC_ETH = ethers.utils.formatEther(amountsOutUSDC_ETH[1].toString()); const arbitrageOpportunity = parseFloat(humanReadableAmountETH_DAI) * parseFloat(humanReadableAmountDAI_USDC) * parseFloat(humanReadableAmountUSDC_ETH) ; // Terminal outputs / logs console.log('PRICES ON UNISWAP V2 WITH 1 ETH @ ' + formattedDate); console.log(`ETH -> DAI = ${humanReadableAmountETH_DAI} dai`); console.log(`DAI -> USDC = ${humanReadableAmountDAI_USDC} usdc`); const ethprofit = (arbitrageOpportunity * humanReadableAmountUSDC_ETH); const profit = ethprofit <= 0 ? "" : "-"; console.log(`USDC -> ETH = ${(ethprofit).toFixed(6)} eth`); console.log(`PROFIT = ${profit}${(1 - ethprofit)*100 .toFixed(6)}% `); } catch (error) { console.error('Error:', error); } } // Call the main function main(); ``` 现在您需要做的就是将\*"替换为您的 HTTP 端点"\* 替换为您自己的 http 端点 - 这实质上将我们连接到以太坊主网 ``` constprovider = new ethers.providers.JsonRpcProvider( '替换为您的 HTTP 端点'); ``` 可以通过在QuickNode上注册免费帐户来获得此信息-。 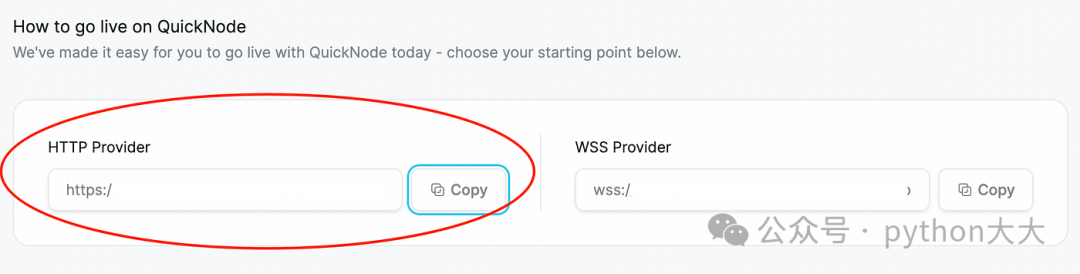 更新后 单击"保存"即可,代码完成...... 第 3 步 --- 运行代码 要运行代码,请在终端中输入以下内容 ``` node uniswapbot.js ``` 该代码只会执行一次,当然,可以定时自动执行代码, 如果代码成功您将看到以下内容 --------------  你可以看到这种交换不会有利可图...... 注意------这不考虑滑点和gas费 =================== 如果互换变得有利可图,策略可能是使用 Furucombo 上的 Aave 闪贷组合来执行此操作。
第 2 步 --- 代码 打开文本编辑器 - 使用Visual Studio Code并打开名为"uniswapbot.js"的文件 粘贴以下代码 ``` // Importing ether's Js const ethers = require('ethers'); // Initialize the router 2 contract const router2Address = '0x7a250d5630b4cf539739df2c5dacb4c659f2488d'; const routerAbi = [ 'function getAmountsOut(uint amountIn, address[] memory path) public view returns (uint[] memory amounts)' ]; // Initialize provider with Infura URL const provider = new ethers.providers.JsonRpcProvider('REPLACE WITH YOUR HTTP ENDPOINT'); // Initialize the router contract const router = new ethers.Contract(router2Address, routerAbi, provider); // Define the swap amount and paths const amountIn = ethers.utils.parseEther('1'); const ethAddress = '0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2'; const daiAddress = '0x6B175474E89094C44Da98b954EedeAC495271d0F'; const usdcAddress = '0xA0b86991c6218b36c1d19D4a2e9Eb0cE3606eB48'; const pathETH_DAI = [ethAddress, daiAddress]; const pathDAI_USDC = [daiAddress, usdcAddress]; const pathUSDC_ETH = [usdcAddress, ethAddress]; // Main function to get the swap quotes async function main() { try { const currentDate = new Date(); const formattedDate = `${currentDate.toLocaleDateString()} ${currentDate.toLocaleTimeString()}`; const amountsOutETH_DAI = await router.getAmountsOut(amountIn, pathETH_DAI); const humanReadableAmountETH_DAI = ethers.utils.formatUnits(amountsOutETH_DAI[1].toString(), 18); const amountsOutDAI_USDC = await router.getAmountsOut(amountsOutETH_DAI[1], pathDAI_USDC); const humanReadableAmountDAI_USDC = ethers.utils.formatUnits(amountsOutDAI_USDC[1].toString(), 6); const amountsOutUSDC_ETH = await router.getAmountsOut(ethers.utils.parseUnits('1', 6), pathUSDC_ETH); // 1 USDC const humanReadableAmountUSDC_ETH = ethers.utils.formatEther(amountsOutUSDC_ETH[1].toString()); const arbitrageOpportunity = parseFloat(humanReadableAmountETH_DAI) * parseFloat(humanReadableAmountDAI_USDC) * parseFloat(humanReadableAmountUSDC_ETH) ; // Terminal outputs / logs console.log('PRICES ON UNISWAP V2 WITH 1 ETH @ ' + formattedDate); console.log(`ETH -> DAI = ${humanReadableAmountETH_DAI} dai`); console.log(`DAI -> USDC = ${humanReadableAmountDAI_USDC} usdc`); const ethprofit = (arbitrageOpportunity * humanReadableAmountUSDC_ETH); const profit = ethprofit <= 0 ? "" : "-"; console.log(`USDC -> ETH = ${(ethprofit).toFixed(6)} eth`); console.log(`PROFIT = ${profit}${(1 - ethprofit)*100 .toFixed(6)}% `); } catch (error) { console.error('Error:', error); } } // Call the main function main(); ``` 现在您需要做的就是将\*"替换为您的 HTTP 端点"\* 替换为您自己的 http 端点 - 这实质上将我们连接到以太坊主网 ``` constprovider = new ethers.providers.JsonRpcProvider( '替换为您的 HTTP 端点'); ``` 可以通过在QuickNode上注册免费帐户来获得此信息-。 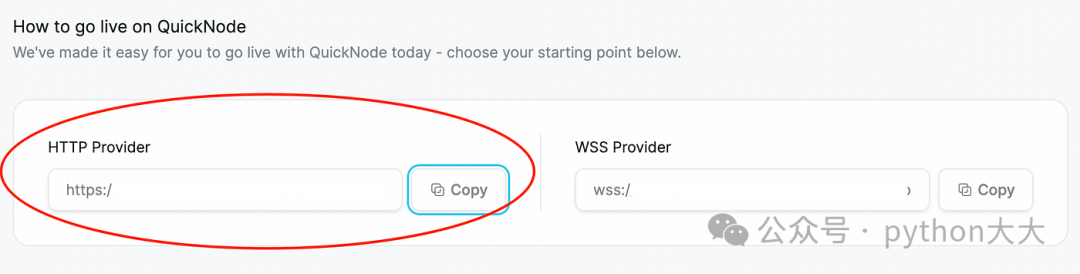 更新后 单击"保存"即可,代码完成...... 第 3 步 --- 运行代码 要运行代码,请在终端中输入以下内容 ``` node uniswapbot.js ``` 该代码只会执行一次,当然,可以定时自动执行代码, 如果代码成功您将看到以下内容 --------------  你可以看到这种交换不会有利可图...... 注意------这不考虑滑点和gas费 =================== 如果互换变得有利可图,策略可能是使用 Furucombo 上的 Aave 闪贷组合来执行此操作。